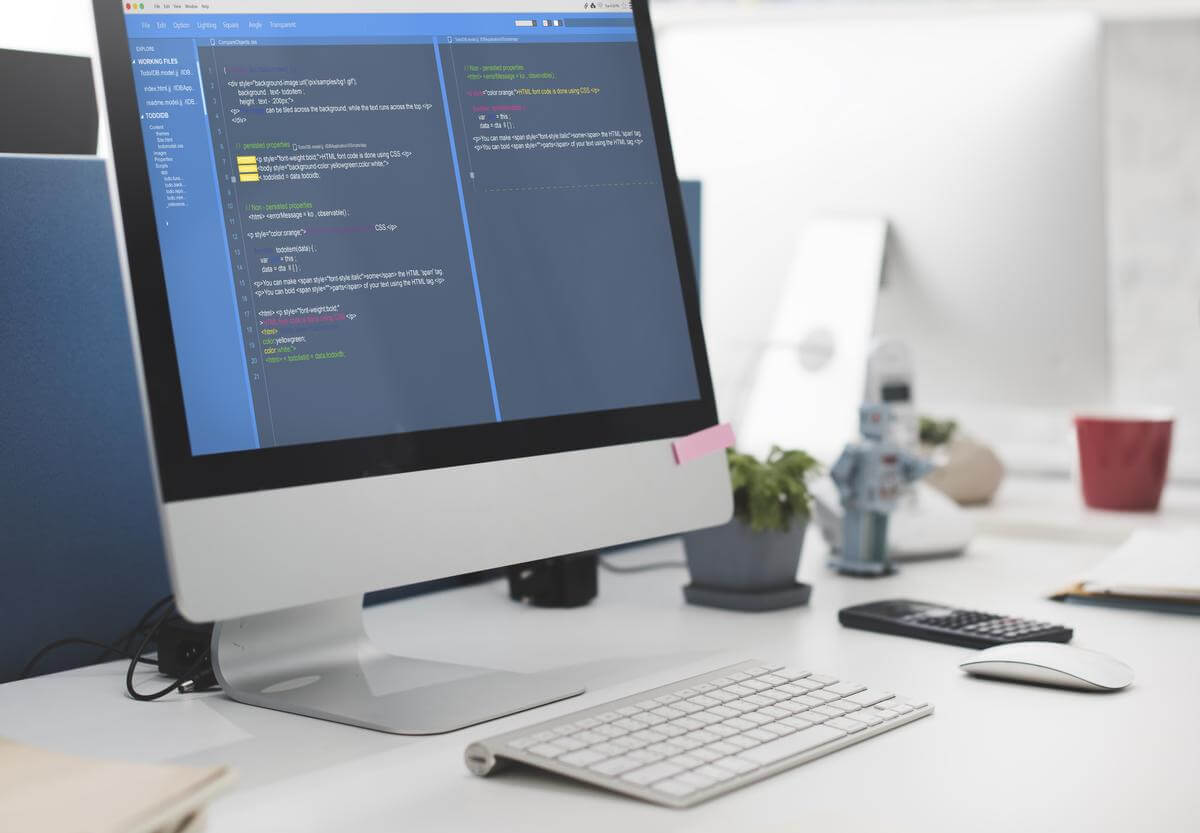
Syntax is one of the most important aspects of any programming language. A programming language’s syntax defines a set of rules that a programmer must follow when writing code. These rules dictate how the code must be structured, what characters can be used, how statements must be terminated, and much more. In essence, syntax is the grammar of a programming language.
Without syntax, it would be impossible for programmers to write programs that could be understood and executed by computers. In fact, syntax is so integral to programming languages that mastering it is often the first step towards becoming a proficient programmer.
Syntax And Its Importance In Programming
Syntax plays a significant role in programming because it is the basis for communicating instructions to a computer. A programming language can be likened to a language that we use to communicate with each other. Without proper syntax, we would not be able to understand each other’s meaning. Similarly, programming languages cannot “understand” code that does not have proper syntax.
At a higher level, proper syntax makes it easier for programmers to create and read code. Since syntax establishes a consistent set of rules, programmers can more easily identify errors, patterns, and best practices. When everyone follows the same syntax rules, it provides a more cohesive programming experience.
Syntax In Common Programming Languages
Syntax varies from one programming language to another. Some programming languages, like python and perl, emphasize “readability” and use whitespace to delimit code blocks. Others, like c++, use curly braces to delimit code blocks and require specific syntax for statements.
Some common programming languages are:
- python
- java
- c++
Specifics Of Syntax In Python
Python uses whitespace to denote code blocks. This means that indentation is mandatory, and the number of spaces or tabs used dictates the hierarchy of the code. In python, if statements and loops are followed by a colon (:), and the subsequent code block must be indented. Variables are assigned using the equals sign (=).
An example of python syntax is:
“`
If x > 0:
Print(“x is positive”)
“`
This code first tests whether the variable `x` is greater than 0. If it is, the code block following the if statement (which consists of a print statement) will be executed.
Specifics Of Syntax In Java
Java syntax is based on c++, with some additional features. Java is considered a strongly typed language, which means that variables must be explicitly declared with a specific data type. Java uses curly braces to delimit code blocks, and a semicolon (;) is used to terminate statements.
An example of java syntax for declaring a variable is:
“`
Int x = 5;
String message = “hello, world!”;
“`
This code defines two variables: an integer variable named `x` with a value of 5, and a string variable named `message` with a value of “hello, world!”
Specifics Of Syntax In C++
C++ syntax is also based on c, with a few notable differences. Like java, c++ is also a strongly typed language. C++ uses curly braces to delimit code blocks, and a semicolon (;) is used to terminate statements. C++ allows for the use of pointers, which are variables that store memory addresses.
An example of c++ syntax for a for loop is:
“`
For (int i = 0; i < 10; i++) { Std::cout << i << “\n”; } “` This code creates a for loop that iterates over the values from 0 to 9, and outputs each value to the console.
Syntax And Programming Paradigms
Programming paradigms refer to the different approaches that programmers can take when solving problems using code. Each programming paradigm has its own set of syntax rules that dictate how code must be structured. Some common programming paradigms include:
- procedural programming
- object-oriented programming
- functional programming
Each of these paradigms has its own syntax rules, which allow programmers to write code that conforms to the philosophy of the paradigm. For example, object-oriented programming languages, like java and python, use syntax that facilitates the creation of objects and classes. Functional programming languages, like lisp and haskell, emphasize the use of pure functions and recursion.
The Role Of Syntax In Code Development
Syntax is an essential part of code development. Without syntax, code cannot be executed by a computer. Additionally, proper syntax makes code easier to read, maintain, and debug. When code is written with consistent syntax, it provides a framework that makes it easier for other programmers to understand the code and contribute to it.
Syntax also has implications for code performance. In some programming languages, inefficient syntax can lead to code that executes more slowly. Therefore, it is often essential to write code that follows best practices and efficient syntax rules to ensure that it is both readable and performant.
On Syntax In Programming Languages
As we’ve seen, syntax is a critical aspect of any programming language. From python and java to c++ and beyond, syntax rules establish a consistent framework for how code is written, and how it can be understood and executed by a computer. Syntax makes code more accessible to other programmers, enables efficient performance, and facilitates the development of software that solves complex problems.
As you learn more about programming, syntax will be one of the first things you master. Investing time and effort into mastering proper syntax rules for specific languages will pay dividends in code readability, maintainability, and ultimately, software performance.